Table of Contents
- Getting Started
- EO.Pdf
- Overview
- Installation and Deployment
- Using HTML to PDF
- Using PDF Creator
- Using PDF Creator
- Getting Started
- Advanced Formatting Techniques
- Interactive Features
- Low Level Content Objects
- Working with Existing PDF Files
- Using in Web Application
- Advanced Topics
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Common Topics
- Reference
Creating Fill In Form |
Overview
EO.Pdf provides a set of input field objects that you can add into the content tree. Those input fields are rendered as PDF form field when the contents are rendered.
Quick Start Sample
The following code demonstrates how to create a small form with two text boxes asking for first name and last name.
//Create the root content AcmContent root = new AcmContent(); //Create the "First Name" paragraph AcmParagraph p = new AcmParagraph(new AcmText("First Name: ")); root.Children.Add(p); //Create the "First Name" text box AcmTextBox tbFirstName = new AcmTextBox(); //Set the textbox size tbFirstName.Style.Width = 2f; tbFirstName.Style.Height = 0.2f; //Move the textbox slightly downwards tbFirstName.Style.OffsetY = 0.04f; //Set field name tbFirstName.Name = "first_name"; //Add the textbox into the content tree p.Children.Add(tbFirstName); //Create the "Last Name" paragraph p = new AcmParagraph(new AcmText("Last Name: ")); root.Children.Add(p); //Create the "Last Name" text box AcmTextBox tbLastName = new AcmTextBox(); //Set the textbox size tbLastName.Style.Width = 2f; tbLastName.Style.Height = 0.2f; //Move the textbox slightly downwards tbLastName.Style.OffsetY = 0.04f; //Set field name tbLastName.Name = "last_name"; //Add the textbox into the content tree p.Children.Add(tbLastName);
The above code produces the follow result:
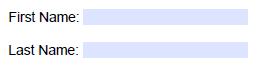
Supported Input Field Types
EO.Pdf currently supports the following input field types:
Field Type | Remarks |
---|---|
AcmTextBox | Create a textbox. |
AcmCheckBox | Create a checkbox. |
AcmListBox | Create a list box. |
AcmRadioButton | Create a radio button. |
Because all input field types derive from AcmContent, they are used the same way as any other content types -- just add them into the content tree and then render the content tree. However a few extra steps are usually needed for input fields:
- Explicit Width and Height are usually needed (default size are used if Width and Height are not set). Unlike most inline contents that can determine its own size based on the content (for example, an AcmText can determine its size based on the text and font), an input field usually can not derive its size based on the content --- for example, an AcmTextBox would not know its appropriate size when the textbox is completely empty;
- Very often you need to set OffsetY to a negative value so that the input field and the text before/after the field would appear to be on the same base line;
- Each input field usually has a unique Name that identify the field in the form for data exchange purposes;