Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Multi-Selection |
EO.Wpf ListBox supports multi-selection. This section covers the following topics:
Enabling Multi-Selection
To enable multi-selection, set the ListBox's SelectionMode to Multiple or Extended. When this property is set to Multiple, the user can select multiple items without holding down a modifier key. When this property is set to Extended, the user can select multiple items while holding down the SHIFT key. The following code demonstrates how to use this feature:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <eo:ListBox Width="200" HorizontalAlignment="Left" SelectionMode="Multiple"> <eo:ListBoxItem>Item 1</eo:ListBoxItem> <eo:ListBoxItem>Item 2</eo:ListBoxItem> <eo:ListBoxItem>Item 3</eo:ListBoxItem> <eo:ListBoxItem>Item 4</eo:ListBoxItem> <eo:ListBoxItem>Item 5</eo:ListBoxItem> </eo:ListBox> </StackPanel> </Window>
The above code produces the following result:
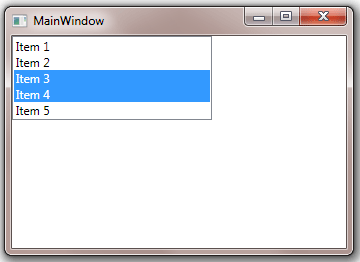
Displaying CheckBox
You can set ShowCheckBoxes to true to display a checkbox for every item. When ShowCheckBoxes is set to true, the ListBox displays a default HeaderTemplate that contains a single checkbox through which you can select/unselect all items. The following code demonstrates this behavior:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <eo:ListBox Width="200" HorizontalAlignment="Left" ShowCheckBoxes="True"> <eo:ListBoxItem>Item 1</eo:ListBoxItem> <eo:ListBoxItem>Item 2</eo:ListBoxItem> <eo:ListBoxItem>Item 3</eo:ListBoxItem> <eo:ListBoxItem>Item 4</eo:ListBoxItem> <eo:ListBoxItem>Item 5</eo:ListBoxItem> </eo:ListBox> </StackPanel> </Window>
The above code provides the following result:
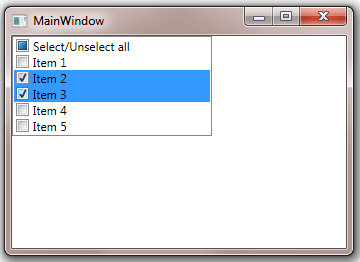
You can set ShowHeader to false to turn off the default header.