Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Validating User Input |
EO.Wpf MaskedEdit can automatically highlight a segment if user input in that segment is invalid. To use this feature, simply set the MaskedEdit's AutoHighlightIfInvalid to true. The following sample demonstrates this feature:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" xmlns:g="clr-namespace:System.Globalization;assembly=mscorlib" Title="MainWindow" Height="250" Width="350"> <StackPanel Margin="10"> <eo:MaskedEdit PromptChar="_" AutoHighlightIfInvalid="True" Width="60" HorizontalAlignment="Left"> <eo:MaskMaskedEditSegment Mask="AAAA"></eo:MaskMaskedEditSegment> <eo:StaticMaskedEditSegment Text="-"></eo:StaticMaskedEditSegment> <eo:NumericMaskedEditSegment IsRequired="True" Digits="3"></eo:NumericMaskedEditSegment> </eo:MaskedEdit> </StackPanel> </Window>
Run the above code, enter a single letter in the first segment, then tab into the second segment, you will see the first segment is highlighted in red:
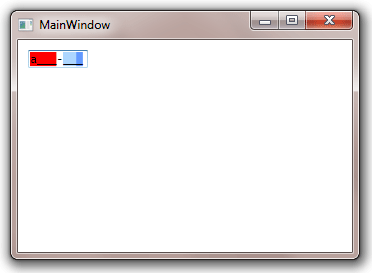
The segment is highlighted in red because the segment's Mask is "AAAA", which represents 4 required letters. Since user has only entered one, it is considered as invalid. Likewise, if user does not enter anything at all in the second segment (the numeric segment), that segment will be highlighted as red as well because that segment's IsRequired is set to True.
You can customize the highlight color by using a different ErrorBrush. For example, the following code uses yellow to highlight error:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" xmlns:g="clr-namespace:System.Globalization;assembly=mscorlib" Title="MainWindow" Height="250" Width="350"> <StackPanel Margin="10"> <eo:MaskedEdit PromptChar="_" AutoHighlightIfInvalid="True" Width="60" HorizontalAlignment="Left" ErrorBrush="Yellow"> <eo:MaskMaskedEditSegment Mask="AAAA"></eo:MaskMaskedEditSegment> <eo:StaticMaskedEditSegment Text="-"></eo:StaticMaskedEditSegment> <eo:NumericMaskedEditSegment IsRequired="True" Digits="3"></eo:NumericMaskedEditSegment> </eo:MaskedEdit> </StackPanel> </Window>
Sometimes you may need to clear the error highlight. For example, if you have a form, you may have a "Reset" button that will allow user to start over. In that case you must clear all existing errors highlights. You can call ResetErrorHighlights method for this purpose.