Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Advanced Content |
EO.Wpf ProgressBar can display additional content on top of the ProgressBar. This section covers the following topic:
Displaying Current Value
To display the current value, simply set the ProgressBar's ShowContent to true:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" xmlns:g="clr-namespace:System.Globalization;assembly=mscorlib" Title="MainWindow" Height="250" Width="350"> <StackPanel Margin="10"> <eo:ProgressBar Height="20" Minimum="0" Maximum="100" Value="30" ShowContent="True"></eo:ProgressBar> </StackPanel> </Window>
The above code produces the following result:
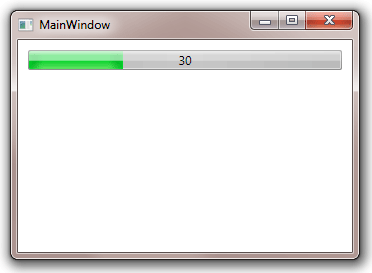
Displaying Current Percentage
You can set the ProgressBar's IsPercent to true to display the percentage value. Note that if the ProgressBar's Minimum is 0 and Maximum is 100, which are the default values, then the percentage value will be the same as the ProgressBar's Value.
If you also wish to display the percentage sign, you can set ContentStringFormat to "{}{0}%". Note two pair of curly brackets is needed because by default curly bracket introduces a data binding expression.
The following XAML demonstrates this feature:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" xmlns:g="clr-namespace:System.Globalization;assembly=mscorlib" Title="MainWindow" Height="250" Width="350"> <StackPanel Margin="10"> <eo:ProgressBar Height="20" Minimum="0" Maximum="200" Value="30" ShowContent="True" IsPercent="True" ContentStringFormat="{}{0}%"></eo:ProgressBar> </StackPanel> </Window>
The above code produces the following result:
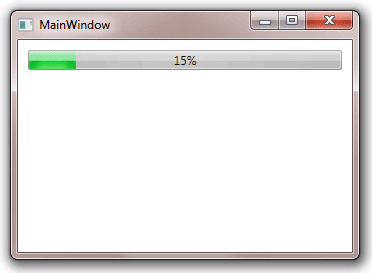
Using Content Templates
You can use ContentTemplate property to display complex information on the ProgressBar. The following XAML demonstrates this feature:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" xmlns:g="clr-namespace:System.Globalization;assembly=mscorlib" Title="MainWindow" Height="250" Width="350"> <StackPanel Margin="10"> <eo:ProgressBar VerticalAlignment="Center" Height="23" Minimum="0" Maximum="200" Value="135" ShowContent="True"> <eo:ProgressBar.ContentTemplate> <DataTemplate> <StackPanel Orientation="Horizontal"> <TextBlock VerticalAlignment="Center" Text="Downloaded " /> <TextBlock VerticalAlignment="Center" Text="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:ProgressBar}, Path=DisplayValue}" /> <TextBlock VerticalAlignment="Center" Text=" files out of " /> <TextBlock VerticalAlignment="Center" Text="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:ProgressBar}, Path=Maximum}" /> </StackPanel> </DataTemplate> </eo:ProgressBar.ContentTemplate> </eo:ProgressBar> </StackPanel> </Window>
The above code produces the following result:
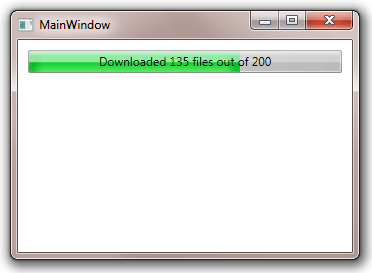