Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Dynamic TreeView from Data Source |
EO.Wpf TreeView derives from ItemsControl, so you can uses the ItemsControl's ItemsSource property to populate the TreeView. Additionally, you can also use HierarchicalDataTemplate to populate the TreeView hierarchically.
Populating from a string array
The following sample uses an array for ItemsSource:
//Populate the items from an array TreeView1.ItemsSource = new string[]{"Item 1", "Item 2", "Item 3", "Item 4", "Item 5"};
The above code produces the following result:
Populating from complex objects
The object in the list can be complex object. For example, the following sample demonstrates how to use "Celebrity" object to populate the TreeView:
//Define a celebrity with a name and a picture internal class Celebrity : INotifyPropertyChanged { private string m_szName; private string m_szImageUri; public event PropertyChangedEventHandler PropertyChanged; public Celebrity(string name, string imageUri) { m_szName = name; m_szImageUri = imageUri; } public string Name { get { return m_szName; } set { if (m_szName != value) { m_szName = value; OnPropertyChanged("Name"); } } } public string ImageUri { get { return m_szImageUri; } } public ImageSource Image { get { Uri uri = new Uri("pack://application:,,,/Images/Celebrities/" + m_szImageUri); return new BitmapImage(uri); } } private void OnPropertyChanged(string name) { PropertyChangedEventHandler handler = PropertyChanged; if (handler != null) { handler(this, new PropertyChangedEventArgs(name)); } } } //Populate the TreeView Celebrity[] popularSingers = new Celebrity[] { new Celebrity("Carly Rae Jepsen", "Carly_Rae_Jepsen.gif"), new Celebrity("Cher Lloyd", "Cher_Lloyd.gif"), new Celebrity("Chris Brown", "Chris_Brown.gif"), new Celebrity("Flo Rida", "Flo_Rida.gif"), new Celebrity("Justin Bieber", "Justin_Bieber.gif"), new Celebrity("Katy Perry", "Katy_Perry.gif"), new Celebrity("Kelly Clarkson", "Kelly_Clarkson.gif"), }; TreeView1.ItemsSource = popularSingers;
Note in the above sample, it is necessary to set the TreeView's ItemTemplate property in order to display the item correctly. In the above example, the ItemTemplate contains a TextBlock element to display the person's name and a Bitmap element to display the person's picture.
The above code produces the following result:
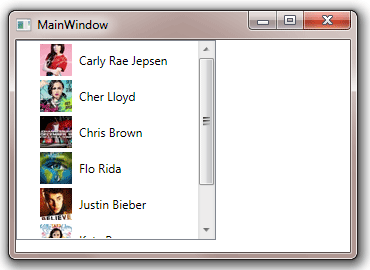
Populating from hierarchical data source
All the above examples only populate one level of items. The following example demonstrates how to populate multiple-level items with HierarchicalDataTemplate. This sample also uses the Celebrity class defined in the previous sample, but also defines a new CelebrityCategory class:
internal class CelebrityCategory : INotifyPropertyChanged { private string m_szName; private string m_szImageUri; private Celebrity[] m_Celebrities; public event PropertyChangedEventHandler PropertyChanged; public CelebrityCategory(string name, string imageUri, Celebrity[] celebrities) { m_szName = name; m_szImageUri = imageUri; m_Celebrities = celebrities; } public string Name { get { return m_szName; } set { if (m_szName != value) { m_szName = value; OnPropertyChanged("Name"); } } } public string ImageUri { get { return m_szImageUri; } set { m_szImageUri = value; } } public ImageSource Image { get { Uri uri = new Uri("pack://application:,,,/Images/" + m_szImageUri); return new BitmapImage(uri); } } public Celebrity[] Celebrities { get { return m_Celebrities; } } private void OnPropertyChanged(string name) { PropertyChangedEventHandler handler = PropertyChanged; if (handler != null) { handler(this, new PropertyChangedEventArgs(name)); } } }
The above code produces the following result:
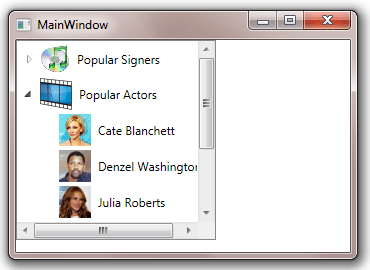
In the above code, the top level items source is a list of CelebrityCategory objects. However because the TreeView's ItemTemplate is a HierarchicalDataTemplate and the HierarchicalDataTemplate's ItemsSource is bind to the CelebrityCategory's Celebrities property (which provides a list of celebrities for that category), each top level item is further populated with a list of child items based on the HierarchicalDataTemplate's ItemTemplate, with each child item representing a celebrity. In this sample the top level item's ItemTemplate (the content of the HierarchicalDataTemplate) and the child item's ItemTemplate (the content of HierarchicalDataTemplate.ItemTemplate) are very similar. However in reality they can be very different.