Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- Overview
- Installation & Deployement
- EO.Web ToolTip
- EO.Web Rating
- EO.Web Slider & RangeSlider
- EO.Web ListBox
- EO.Web ComboBox
- EO.Web Captcha
- EO.Web ASPX To PDF
- EO.Web Slide
- EO.Web Flyout
- EO.Web EditableLabel
- EO.Web ImageZoom
- EO.Web Floater
- EO.Web Downloader
- EO.Web ColorPicker
- EO.Web HTML Editor
- EO.Web File Explorer
- EO.Web SpellChecker
- EO.Web Grid
- EO.Web MaskedEdit
- EO.Web Splitter
- EO.Web Menu
- EO.Web Slide Menu
- EO.Web TabStrip
- EO.Web TreeView
- EO.Web TreeView
- Overview
- Using EO.Web TreeView
- TreeNode and TreeNodeGroup
- Look, Skin and Theme
- Style and Appearance
- Data Binding
- Handling Event
- EO.Web Calendar
- EO.Web Callback
- EO.Web MultiPage
- EO.Web Dialog
- EO.Web AJAXUploader
- EO.Web ProgressBar - Free!
- EO.Web ToolBar - Free!
- EO.WebBrowser
- EO.Wpf
- Common Topics
- Reference
Populating from DataTable, DataView or IDataReader |
Apply to
Overview
EO.Web controls can populate hierarchical navigation item structure from DataTable, DataView or IDataReader.
Use a DataTable to represent hierarchical data
Consider a table with the following data:
Country | State | City | WebSite |
---|---|---|---|
U.S.A. | GA | Atlanta | http://www.atlantaga.gov |
U.S.A. | GA | Savannah | http://www.ci.savannah.ga.us |
U.S.A. | FL | Miami | http://www.ci.miami.fl.us |
U.S.A. | FL | Orlando | http://www.cityoforlando.net |
Canada | ON | Toronto | http://www.city.toronto.on.ca |
SELECT Country.Name, State.Name, City.Name, City.WebSite FROM Country, State, City WHERE Country.ID = State.CountryID and State.ID = City.StateID
After the table is generated as above, to populate the navigation control from the data table, simply set the control's DataSource to this table, and DataFields to "Country|State|City", which specifies the control to use Country as the data field for top level group, State as data field for second level group and City as the data field for the third level group:
<eo:Menu Runat="server" DataFields="Country|State|City"> </eo:Menu>
Note: EO.Web navigation controls also support binding to hierarchical data that uses parent key & child key in one table. For more details, see populating using Parent/Child key relation.
Mapping data field to item's property
When binding to a table, by default data field value in records are assigned to an item's Text.Html property. If you intend to assign data field value to other property, you can define one or more DataBinding objects to customize the mapping between a specific data field and a specific property. The code below maps data field WebSite to NavigateUrl for the City level navigation items:
<eo:Menu Runat="server" DataFields="Country|State|City"> <topgroup> <Bindings> <eo:DataBinding Property="NavigateUrl" DataField="WebSite" Depth="2"> </eo:DataBinding> </Bindings> </topgroup> </eo:Menu>
You can also override the default mapping. The following code uses WebSite instead of City as navigation item text:
<eo:Menu Runat="server" DataFields="Country|State|City"> <topgroup> <Bindings> <eo:DataBinding Property="Text-Html" DataField="WebSite" Depth="2"> </eo:DataBinding> </Bindings> </topgroup> </eo:Menu>
Use Depth to specify which level the mapping should be applied to. Depth begins with 0.
Populating the whole navigation control
EO.Web navigation controls supports populating the whole control from a data source by binding the data source to the control. Simply specify the control's DataSource and DataFields properties, then call the control's DataBind method to construct the control from the data source.
Here's a sample of editting a Menu's Bindings property:
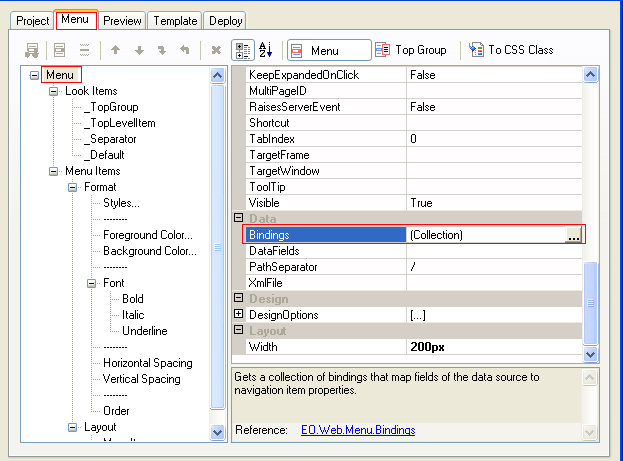
You can define multiple mappings on each level. Level specified by Depth property starts from 0.
Populating the group
EO.Web navigation controls support populating a certain group from a data source. Simply specify the group's DataFields and DataSource properties, then call DataBind method.
Here's a sample of editting a MenuGroup's Bindings property
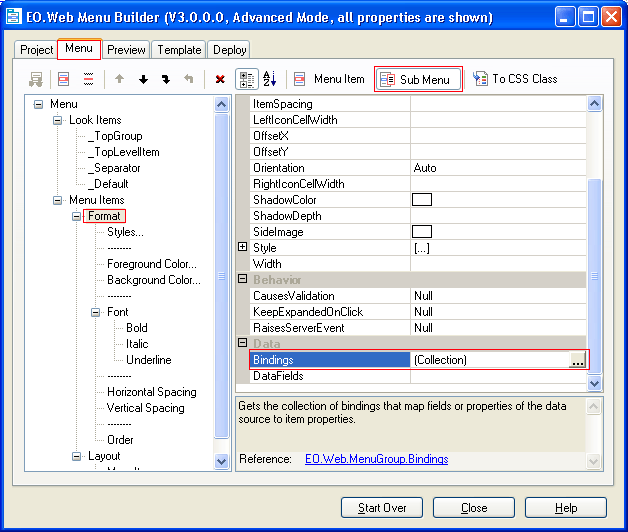
Summary
- Drag an EO.Web navigation control onto the page;
- Prepare the DataTable object (or DataView, IDataReader);
- Set the control or group's DataFields property;
- Optionally, define one or more DataBinding objects on for the control or group;
- Call DataBind method on the control or group.