Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Getting Started |
A docking UI can be created either with XAML or through code, or a combination of both. The first step to create a docking UI is to place a DockContainer control in your XAML:
<Window x:Class="Test.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="Window1" Height="500" Width="600"> <eo:DockContainer> </eo:DockContainer> </Window>
A DockContainer is the root element of a docking UI. The following image demonstrates how a typical DockContainer divides its client area:
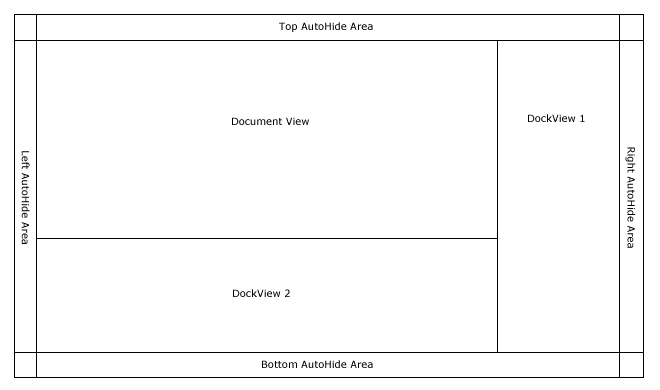
A DockContainer can contain one or more regular dock view or document views. A document view is usually the main document area of a docking UI, while multiple regular dock views provide various docking tool windows. In the above image, it has one document view and two regular dock views.
Both regular dock views and document views are represented by DockView class. A DockView is a document view when its IsDocumentView property is set to true. At runtime, a document view differs from a regular dock view in many different ways. For example, when a document view contains multiple items, it creates a tab strip at the top of the view for user to switch items. A regular dock view also creates a tab strip but the tab strip is created at the bottom of the view.
To create one or more views inside a DockContainer, simply place one or more DockView elements inside the DockContainer: The following XAML creates the layout demonstrated in the above image:
<Window x:Class="Test.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="Window1" Height="500" Width="600"> <eo:DockContainer> <!-- DockView1 docks to the bottom --> <eo:DockView Dock="Right" Width="150"> </eo:DockView> <!-- DockView2 docks to the right --> <eo:DockView Dock="Bottom" Height="150"> </eo:DockView> <!-- The third view is a document view --> <eo:DockView IsDocumentView="True"> </eo:DockView> </eo:DockContainer> </Window>
The above code produces the following result:
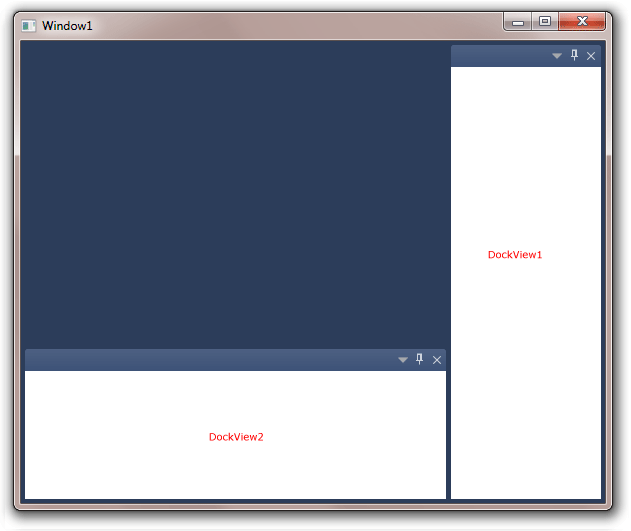
Note a DockView's placement in the DockContainer is determined by its Dock property. For example, in the above sample, the first view (DockView1) is docked to the right, the second view (DockView2) is docked to the bottom, what's left is for the main document view. Because DockView1 is docked to the right first, it occupies the full height, which means DockView2 does not occupies the full width.
If the first view is docked to the bottom, then the bottom view would occupy the full width:
<Window x:Class="Test.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="Window1" Height="500" Width="600"> <eo:DockContainer> <!-- DockView1 docks to the bottom --> <eo:DockView Dock="Bottom" Height="150"> </eo:DockView> <!-- DockView2 docks to the right --> <eo:DockView Dock="Right" Width="150"> </eo:DockView> <!-- The third view is a document view --> <eo:DockView IsDocumentView="True"> </eo:DockView> </eo:DockContainer> </Window>
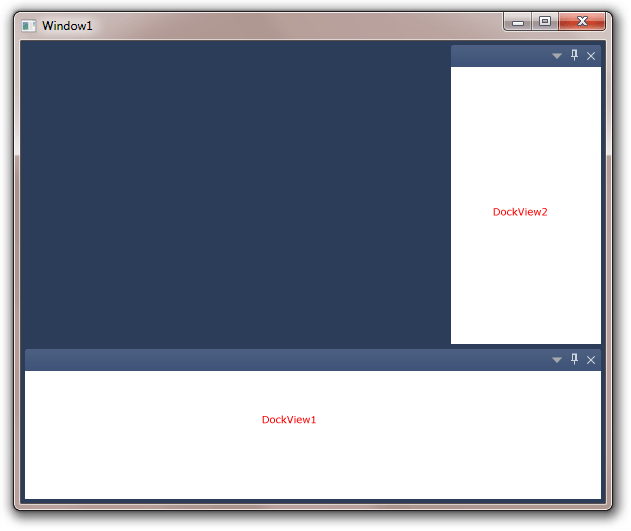
DockView can also be nested. The following XAML creates nested dock views:
<Window x:Class="Test.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="Window1" Height="500" Width="600"> <eo:DockContainer> <!-- This dock view contains two child dock views --> <eo:DockView Dock="Right" Width="150"> <eo:DockView.ChildViews> <!-- The first child dock view --> <eo:DockView Dock="Top"> </eo:DockView> <!-- The second child dock view --> <eo:DockView></eo:DockView> </eo:DockView.ChildViews> </eo:DockView> <!-- DockView2 docks to the right --> <eo:DockView Dock="Bottom" Height="150"> </eo:DockView> <!-- The third view is a document view --> <eo:DockView IsDocumentView="True"> </eo:DockView> </eo:DockContainer> </Window>
The above code produces the following result:
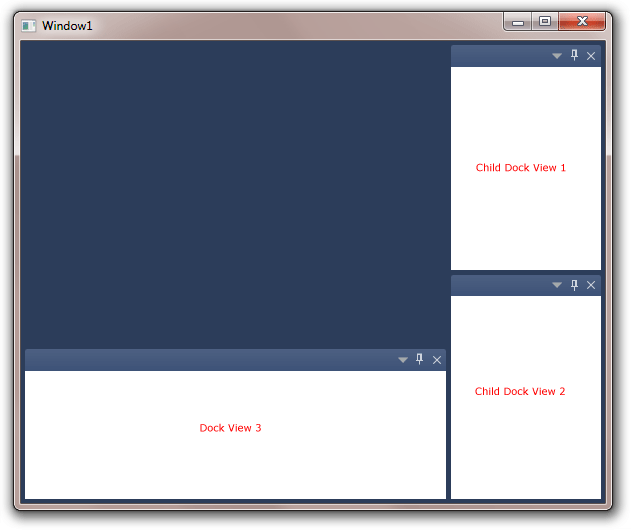
All the views in the above samples are empty. In order for a view to be useful, you must add one or more DockItem into the DockView. The following XAML completes the above XAML with a few DockItems:
<Window x:Class="Test.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="Window1" Height="500" Width="600"> <eo:DockContainer> <!-- DockView1 docks to the right --> <eo:DockView Dock="Right" Width="150"> <eo:DockItem Title="Project"> <TextBlock>Project View UI</TextBlock> </eo:DockItem> <eo:DockItem Title="Toolbox"> <TextBlock>Toolbox View UI</TextBlock> </eo:DockItem> </eo:DockView> <!-- DockView2 docks to the bottom --> <eo:DockView Dock="Bottom" Height="150"> <eo:DockItem Title="Debug"></eo:DockItem> <eo:DockItem Title="Output"></eo:DockItem> <eo:DockItem Title="Watch"></eo:DockItem> </eo:DockView> <!-- The third view is a document view --> <eo:DockView IsDocumentView="True"> <eo:DockItem Title="Document1"> </eo:DockItem> <eo:DockItem Title="Document2"> </eo:DockItem> </eo:DockView> </eo:DockContainer> </Window>
The above code produces the following result:
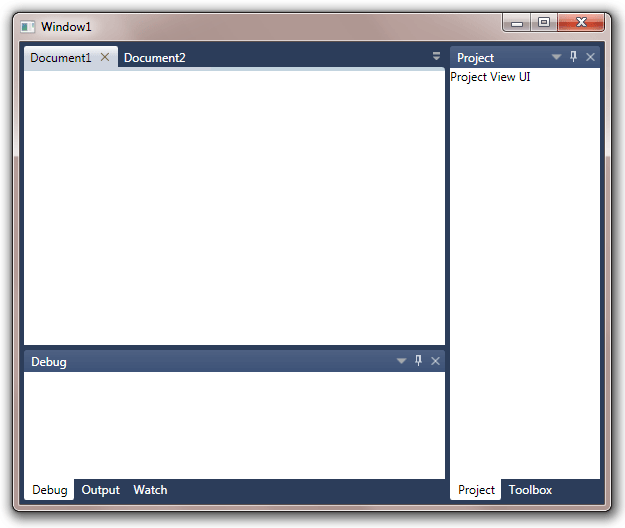
Each DockItem is also a ContentControl, you should place your actual view contents inside the corresponding DockItem.