Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf Gauge
- Using Scales
- Using Gauge Frames
- Creating a Gauge
- Understanding TickBar
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Customizing Needle |
Needle is a type of ValueIndicator that can only be used with CircularScale. At runtime, the CircularScale automatically rotate the Needle to the proper angle so that it points to the Value it represents.
A Needle consists of three parts: The cap, the body, and the tip. The following image demonstrates these parts:
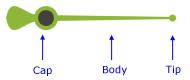
Customizing Cap
You can use CapTemplate to customize the cap of the needle. The following template is used to create the Needle displayed in the above image:
<DataTemplate> <Grid Margin="-12,0,0,0"> <Path Fill="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:Needle}, Path=Background}" Width="35" HorizontalAlignment="Left" Stretch="Fill" Margin="-28,0,0,0"> <Path.Data> <PathGeometry> <PathFigure StartPoint="0,15"> <BezierSegment Point1="5,-25" Point2="20,15" Point3="35,10" /> <LineSegment Point="35,20" /> <BezierSegment Point2="5,55" Point1="20,15" Point3="0,15" /> </PathFigure> </PathGeometry> </Path.Data> </Path> <Ellipse Width="24" Height="24" Fill="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:Needle}, Path=Background}" /> <Ellipse Width="16" Height="16" Fill="#43413C" VerticalAlignment="Center" /> </Grid> </DataTemplate>
Key points of interest:
- The Grid has a negative left margin. This is common practice to define the CapTemplate of a Needle. This is because the actual left margin of the Needle is the center of the scale. Because it's almost always the case that a portion of the needle cap will exceeds the center (to the left if the Needle points to the right), so a negative margin is often used for this purpose;
- The above sample uses RelativeSource binding to apply the Needle's background;
In addition to CapTemplate, CapStyle can also be used to customize the cap. The target type of CapStyle is ContentControl. CapStyle is often used to apply z-index for the cap.
Customizing Body
The body of the Needle can be customized through three properties: RearWidth, TipWidth and RearOffset.
RearWidth and TipWidth can be used to customize the size (thickness) of the center end and the tip end of the body respecitvely. Typically, RearWidth would have a bigger value than TipWidth. Set TipWidth to a small value, for example, 1, to create a sharp tip.
RearOffset can be used to reserve some space between the center of the needle cap and the left edge of the needle body. This property is not commonly used.
Customizing Tip
You can use TipTemplate to customize the tip of the Needle. TipTemplate is very similar to CapTemplate, except that CapTemplate aligns to the left and TipTemplate aligns to the right. The following XAML defines the small round tip shown in the above picture:
<DataTemplate> <Ellipse Width="7" Height="7" Fill="#8DB739"></Ellipse> </DataTemplate>
Setting Needle Length
Use the Needle control's Width property to customize the needle length. This is because the Needle is always customized as a horizontal needle pointing to the right first, then rotated to the desired angle.
Complete Example
The following XAML code is a complete example:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="300" Width="350"> <Grid eo:ThemeManager.SkinName="Clean"> <eo:CircularScale Minimum="0" Maximum="60" StartAngle="270" EndAngle="270" ShowFirst="False"> <eo:CircularScale.RingDefinitions> <eo:RingDefinition RadiusX="100" RadiusY="80"></eo:RingDefinition> <eo:RingDefinition RadiusX="120" RadiusY="100"></eo:RingDefinition> </eo:CircularScale.RingDefinitions> <eo:CircularScale.MajorTickOptions> <eo:MajorCircularTickOptions Ring="1"></eo:MajorCircularTickOptions> </eo:CircularScale.MajorTickOptions> <eo:CircularScale.MinorTickOptions> <eo:MinorCircularTickOptions Ring="1"></eo:MinorCircularTickOptions> </eo:CircularScale.MinorTickOptions> <eo:CircularScale.ValueIndicators> <eo:Needle Value="15" TipWidth="2"> <eo:Needle.CapTemplate> <DataTemplate> <Grid Margin="-12,0,0,0"> <Path Fill="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:Needle}, Path=Background}" Width="35" HorizontalAlignment="Left" Stretch="Fill" Margin="-28,0,0,0"> <Path.Data> <PathGeometry> <PathFigure StartPoint="0,15"> <BezierSegment Point1="5,-25" Point2="20,15" Point3="35,10" /> <LineSegment Point="35,20" /> <BezierSegment Point2="5,55" Point1="20,15" Point3="0,15" /> </PathFigure> </PathGeometry> </Path.Data> </Path> <Ellipse Width="24" Height="24" Fill="{Binding RelativeSource={RelativeSource FindAncestor, AncestorType=eo:Needle}, Path=Background}" /> <Ellipse Width="16" Height="16" Fill="#43413C" VerticalAlignment="Center" /> </Grid> </DataTemplate> </eo:Needle.CapTemplate> <eo:Needle.TipTemplate> <DataTemplate> <Ellipse Width="7" Height="7" Fill="#8DB739"></Ellipse> </DataTemplate> </eo:Needle.TipTemplate> </eo:Needle> </eo:CircularScale.ValueIndicators> </eo:CircularScale> </Grid> </Window>
The above code produces the following result:
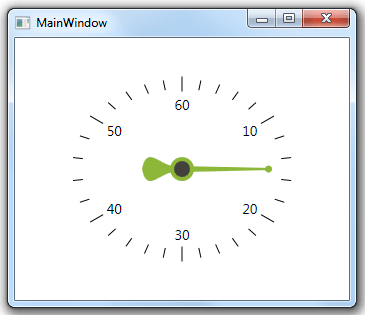