Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Using Menu Icon |
You can set the MenuItem's Icon property to display an icon for the menu. The following XAML demonstrates how to use this feature:
XAML
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <eo:Menu IsMainMenu="True"> <eo:MenuItem Header="_File"> <eo:MenuItem Header="Open"> <eo:MenuItem.Icon> <eo:Bitmap Source="OpenFile.png" /> </eo:MenuItem.Icon> </eo:MenuItem> <eo:MenuItem Header="Exit" /> </eo:MenuItem> <eo:MenuItem Header="_Edit" /> <eo:MenuItem Header="_View" /> <eo:MenuItem Header="_Window" /> <eo:MenuItem Header="_Help" /> </eo:Menu> </StackPanel> </Window>
The above code produces the following result:
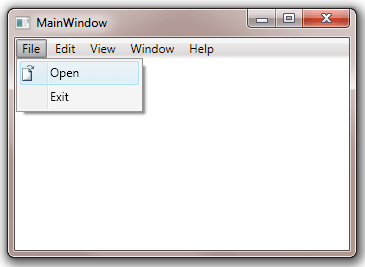
The Icon property is of Object type, so it does not necessarily to be an image. For example, the following sample uses Rectangle object with different background color for the menu item icon:
XAML
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <StackPanel> <eo:Menu IsMainMenu="True"> <eo:MenuItem Header="_Colors"> <eo:MenuItem Header="Red"> <eo:MenuItem.Icon> <Rectangle Fill="Red" Width="16" Height="16"></Rectangle> </eo:MenuItem.Icon> </eo:MenuItem> <eo:MenuItem Header="Green"> <eo:MenuItem.Icon> <Rectangle Fill="Green" Width="16" Height="16"></Rectangle> </eo:MenuItem.Icon> </eo:MenuItem> <eo:MenuItem Header="Blue"> <eo:MenuItem.Icon> <Rectangle Fill="Blue" Width="16" Height="16"></Rectangle> </eo:MenuItem.Icon> </eo:MenuItem> </eo:MenuItem> </eo:Menu> </StackPanel> </StackPanel> </Window>
The above code produces the following result:
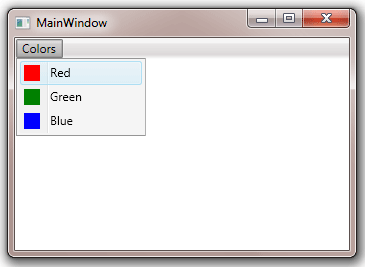
The following sample demonstrates how to set the menu item icon through data binding. The code uses the Celebrity objects.
Celebrity[] popularSingers = new Celebrity[] { new Celebrity("Carly Rae Jepsen", "Carly_Rae_Jepsen.gif"), new Celebrity("Cher Lloyd", "Cher_Lloyd.gif"), new Celebrity("Chris Brown", "Chris_Brown.gif"), new Celebrity("Flo Rida", "Flo_Rida.gif"), new Celebrity("Justin Bieber", "Justin_Bieber.gif"), new Celebrity("Katy Perry", "Katy_Perry.gif"), new Celebrity("Kelly Clarkson", "Kelly_Clarkson.gif"), }; Celebrity[] popularActors = new Celebrity[] { new Celebrity("Cate Blanchett", "Cate_Blanchett.gif"), new Celebrity("Denzel Washington", "Denzel_Washington.gif"), new Celebrity("Julia Roberts", "Julia_Roberts.gif"), new Celebrity("Merly Streep", "Meryl_Streep.gif"), new Celebrity("Tom Cruise", "Tom_Cruise.gif"), new Celebrity("Tom Hanks", "Tom_Hanks.gif"), }; CelebrityCategory[] categories = new CelebrityCategory[] { new CelebrityCategory("Popular Signers", "music.png", popularSingers), new CelebrityCategory("Popular Actors", "movie.png", popularActors), }; Menu1.ItemsSource = categories;