Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Creating Static Menu |
The following XAML demonstrates how to define a static menu items with an EO.Wpf Menu control:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="350" Width="525"> <StackPanel> <eo:Menu IsMainMenu="True"> <eo:MenuItem Header="_File" /> <eo:MenuItem Header="_Edit" /> <eo:MenuItem Header="_View" /> <eo:MenuItem Header="_Window" /> <eo:MenuItem Header="_Help" /> </eo:Menu> </StackPanel> </Window>
The above code produces the following result:
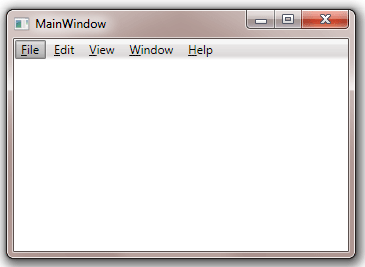
The above code also demonstrates the following features:
- Use "_" to add an underscore to the menu item text. For example, "_File" underscores letter "F". Note the underscore is only displayed when the menu is active;
- IsMainMenu is set to true. This activates the menu if the user presses F10 or the ALT key.
Each menu item can have sub menu items. The following XAML defines two sub menu items for the "File" menu item:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="350" Width="525"> <StackPanel> <eo:Menu IsMainMenu="True"> <eo:MenuItem Header="_File"> <eo:MenuItem Header="_New" Click="File_New_Click" /> <eo:MenuItem Header="_Close" Click="File_Exit_Click" /> </eo:MenuItem> <eo:MenuItem Header="_Edit" /> <eo:MenuItem Header="_View" /> <eo:MenuItem Header="_Window" /> <eo:MenuItem Header="_Help" /> </eo:Menu> </StackPanel> </Window>
The above code produces the following result:
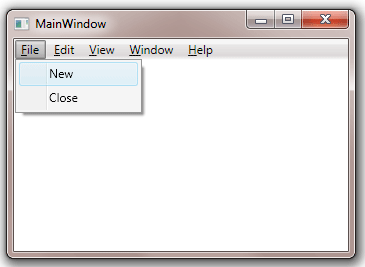
The above code also demonstrates how to handle menu item click event. For example, the File_Close event handler can contain the following code to close the window when the item is clicked:
private void File_Exit_Click(object sender, RoutedEventArgs e) { //Close the window Close(); }
Alternately, you can also use Command property to bind the menu item to a command. The following code also demonstrates how to add a keyboard shortcut to the menu item:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="350" Width="525"> <Window.InputBindings> <KeyBinding Key="X" Modifiers="Alt" Command="ApplicationCommands.Close" /> </Window.InputBindings> <Window.CommandBindings> <CommandBinding Command="ApplicationCommands.Close" Executed="File_Exit_Click"></CommandBinding> </Window.CommandBindings> <StackPanel> <eo:Menu x:Name="Menu1" IsMainMenu="True"> <eo:MenuItem Header="_File"> <eo:MenuItem Header="_New" /> <eo:MenuItem IsSeparator="True"></eo:MenuItem> <eo:MenuItem Header="Close" Command="ApplicationCommands.Close" InputGestureText="Alt+X"></eo:MenuItem> </eo:MenuItem> <eo:MenuItem Header="_Edit" /> <eo:MenuItem Header="_View" /> <eo:MenuItem Header="_Window" /> <eo:MenuItem Header="_Help" /> </eo:Menu> </StackPanel> </Window>
The above code produces the following result:
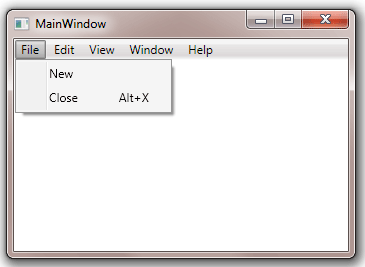
The above sample also demonstrated how to create a separator by setting a MenuItem's IsSeparator to true.