Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Dynamic ComboBox from Data Source |
EO.Wpf ComboBox derives from ItemsControl, so you can uses the ItemsControl's ItemsSource property to populate the ComboBox. This topic covers the following scenarios:
Populating from a string array
The following sample uses an array for ItemsSource:
//Populate the items from an array ComboBox1.ItemsSource = new string[]{"Item 1", "Item 2", "Item 3", "Item 4", "Item 5"};
The above code produces the following result:
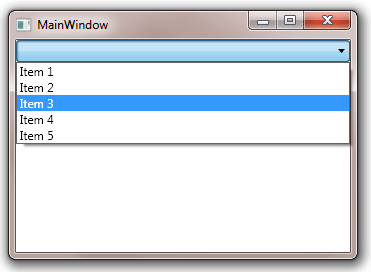
ItemsSource takes any objects that implements IEnumerable.
The following code uses a List
The object in the list can be complex object. For example, the following sample
demonstrates how to use the Celebrity object
to populate the ComboBox:
Note in the above sample, it is necessary to set the ComboBox's
ItemTemplate property in order to
display the item correctly. In the above example, the ItemTemplate contains
a single TextBlock element. You can customize this template to customize
the content of each item. For example, the following code displays the person's
image in the ComboBox:
The above code produces the following result:
//Build the item list
List<string> items = new List<string>();
items.Add("Item 1");
items.Add("Item 2");
items.Add("Item 3");
items.Add("Item 4");
items.Add("Item 5");
//Populate the ComboBox from the item list
ComboBox1.ItemsSource = items;
Populating from a list of complex objects
Celebrity[] popularSingers = new Celebrity[]
{
new Celebrity("Carly Rae Jepsen", "Carly_Rae_Jepsen.gif"),
new Celebrity("Cher Lloyd", "Cher_Lloyd.gif"),
new Celebrity("Chris Brown", "Chris_Brown.gif"),
new Celebrity("Flo Rida", "Flo_Rida.gif"),
new Celebrity("Justin Bieber", "Justin_Bieber.gif"),
new Celebrity("Katy Perry", "Katy_Perry.gif"),
new Celebrity("Kelly Clarkson", "Kelly_Clarkson.gif"),
};
ComboBox1.ItemsSource = popularSingers;
<Window x:Class="Test.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:eo="http://schemas.essentialobjects.com/wpf/"
Title="MainWindow" Height="250" Width="350">
<StackPanel>
<eo:ComboBox x:Name="ComboBox1" Width="250" HorizontalAlignment="Left">
<eo:ComboBox.ItemTemplate>
<DataTemplate>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
<eo:Bitmap Grid.Column="0" Source="{Binding Image}" Margin="2"></eo:Bitmap>
<TextBlock Grid.Column="1" Text="{Binding Name}" VerticalAlignment="Center" Margin="5"></TextBlock>
</Grid>
</DataTemplate>
</eo:ComboBox.ItemTemplate>
</eo:ComboBox>
</StackPanel>
</Window>