Table of Contents
- Getting Started
- EO.Pdf
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Overview
- Installation & Deployement
- Skin & Theme
- Common Taskes and Services
- EO.Wpf Buttons
- EO.Wpf Calendar & DatePicker
- EO.Wpf ComboBox
- EO.Wpf DockView
- EO.Wpf Gauge
- EO.Wpf ListBox
- EO.Wpf Menu
- EO.Wpf MaskedEdit
- EO.Wpf ProgressBar
- EO.Wpf Slider
- EO.Wpf SpinEdit
- EO.Wpf SplitView
- EO.Wpf TabControl
- EO.Wpf TreeView
- EO.Wpf Utility Controls
- EO.Wpf WindowChrome
- Sample Data Objects
- Common Topics
- Reference
Static ComboBox |
The following XAML demonstrates how to define a static ComboBox with an EO.Wpf ComboBox control:
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <eo:ComboBox> <eo:ComboBoxItem>Item 1</eo:ComboBoxItem> <eo:ComboBoxItem>Item 2</eo:ComboBoxItem> <eo:ComboBoxItem>Item 3</eo:ComboBoxItem> <eo:ComboBoxItem>Item 4</eo:ComboBoxItem> <eo:ComboBoxItem>Item 5</eo:ComboBoxItem> </eo:ComboBox> </StackPanel> </Window>
The above code produces the following result:
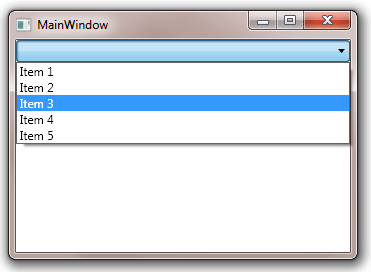
By default, the ComboBox is selection only. If you set the ComboBox's IsEditable to true, then the ComboBox will create a TextBox in the selection box to allow user to enter a value direction (when IsReadOnly is false), or to select and copy selected item text (when IsReadOnly is true):
<Window x:Class="Test.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:eo="http://schemas.essentialobjects.com/wpf/" Title="MainWindow" Height="250" Width="350"> <StackPanel> <eo:ComboBox IsEditable="True"> <eo:ComboBoxItem>Item 1</eo:ComboBoxItem> <eo:ComboBoxItem>Item 2</eo:ComboBoxItem> <eo:ComboBoxItem>Item 3</eo:ComboBoxItem> <eo:ComboBoxItem>Item 4</eo:ComboBoxItem> <eo:ComboBoxItem>Item 5</eo:ComboBoxItem> </eo:ComboBox> </StackPanel> </Window>
The above code produces the following result:
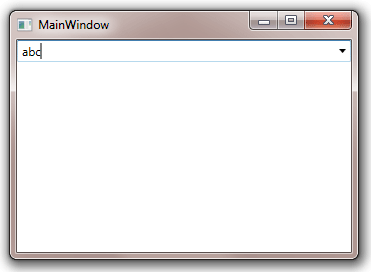
Note user can now directly enter a value in the textbox, and the value does not have to match an existing item. In the above screenshot, "abc" does not match any items, but it is still considered valid and accepted. In this case, the ComboBox's SelectedItem will be null, but its Text will return "abc".
Because a TextBox control is used in this case, user can also select a portion of the selected item text, as demonstrated in the following screenshot:
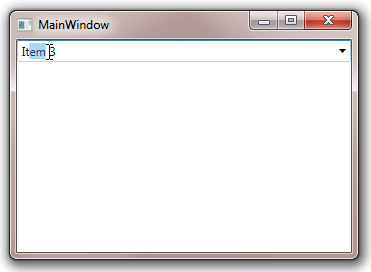
When IsEditable is true, you can also use IsReadOnly to specify whether the TextBox in the selection box is readonly. When the TextBox is readonly, user can not enter any value directly, but they will still be able to select an item from the drop-down, and then select and copy item text as shown in the previous screenshot.